JavaScript ES6: Template Strings
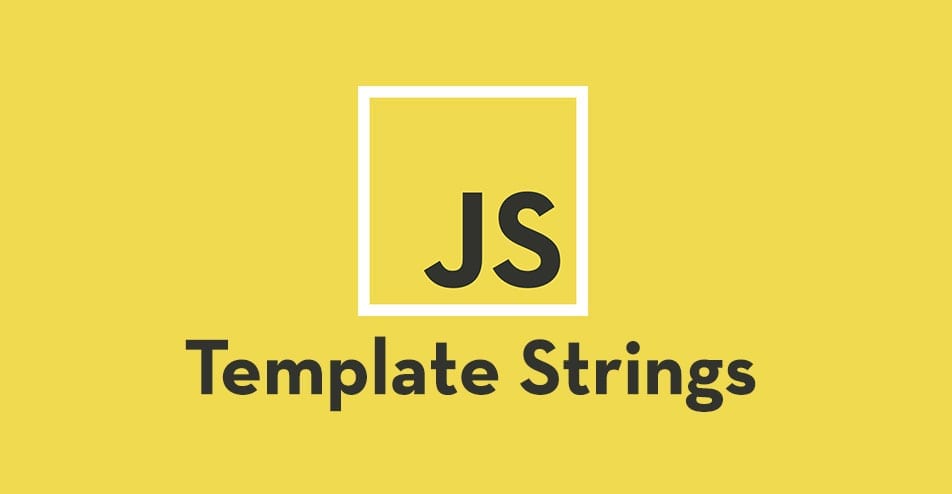
Template strings (or template literals) in JavaScript ES6 is a technique in which you can put variables into strings. Similar to const and let, template strings don’t offer much in the way of new functionality but instead make some quality of life improvements to our syntax.
In ES5, if you want to add variables into a string you need to end the string, concatenate in your variable and start writing your string again.
// ES5
var name = "Jonathan";
var currentDate = new Date().getFullYear();
var sentence = "My name is " + name + " and the year is " + currentDate + ".";
console.log(sentence); // My name is Jonathan and the year is 2020.
In the example above, we are concatenating in two variables (name, currentDate) into a string. As you can see, we have to keep ending the string to add the variables and it’s already getting quite messy with double quotes and plus symbols all over the place. It’s also highly prone to syntax errors and just a pain to have to write out.
Let’s tidy it up a bit with ES6 template strings.
A template string is just a nicer way to join together JavaScript variables with a string.
// ES6
var name = "Jonathan";
var currentDate = new Date().getFullYear();
var sentence = `My name is ${name} and the year is ${currentDate}.`;
console.log(sentence); // My name is Jonathan and the year is 2020.
With template strings, instead of using double or single quotes ,we use back-ticks. Inside the back-ticks, any time we want to add a variable we wrap the variable name in curly brackets and put a dollar sign in front of it.
We’re not limited to just using variable names. Any valid JavaScript expression will work.
// We manipulate the variable
var sentence = `...and the year is ${currentDate * 2}.`; // ...and the year is 4040
// We can ignore the variable entirely and add the date directly into the string
var sentence = `...and the year is ${new Date().getFullYear()}.`; // ...and the year is 2020
Using template strings is a small quality of life improvement. The string written out is more legible than it was when we had to keep using double quotes and plus symbols and is less prone to syntax errors.
Continue Reading
-
Understanding SSL Certificates
SSL certificates are not optional any more, they are critical. Here we help you get a better understanding of what exactly they provide.
-
JavaScript ES6: const and let
Replacing var with const/let is all about increasing clarity in your code and by the end of this article, I’m hoping I will have convinced you to never use var again!
-
Friday wins much coveted Best Website Award at the Spiders
Our award-winning team here at Friday are proud to announce that we have won the much-coveted ‘Best Website’ Award at the annual Spider Awards.
-
WordPress: The Gutenberg Controversy
Gutenberg aims to replace the old CMS text editor WordPress user’s have come to know and love with these new movable blocks. Let’s take a look at what went wrong and if there’s any hope for Gutenberg’s future.