JavaScript ES6: The map() Helper
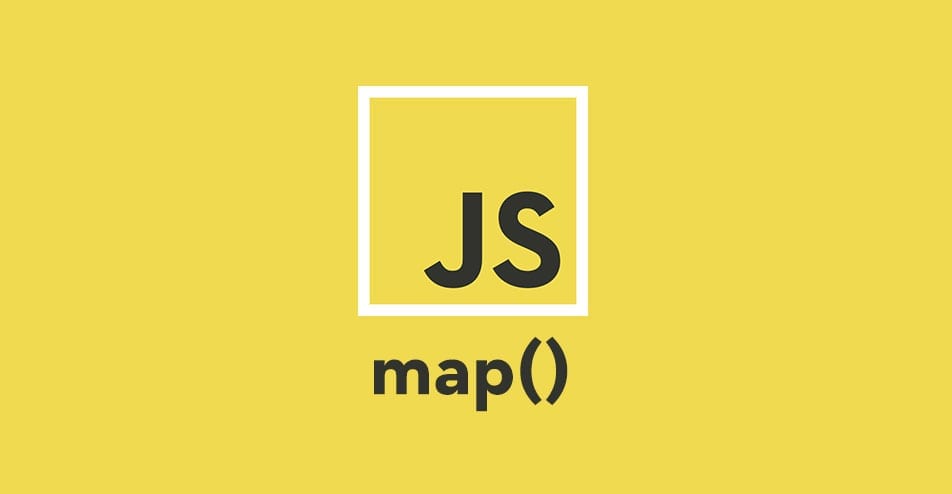
In JavaScript ES6, we use the map() helper when we want to modify a list of data. When map() is finished running, it creates and populates a new array with the results.
In ES5, we would need to use a for loop to iterate over the array and push the results to a previously created empty array.
var numbers = [1, 2, 3];
var doubledNumbers = [];
for( var i = 0; i < numbers.length; i++ ) {
doubledNumbers.push(numbers[i] * 2);
}
console.log(doubledNumbers); // [2, 4, 6]
As with all the ES5 examples, there’s nothing wrong with the for loop. It’s just a bit messy for a number of reasons:
- Lots of different elements of code.
- Prone to typos.
- Semicolons between each element which is uncommon in JS syntax.
- The more logic in a single line of code the more difficult it is for future developers to understand what’s going on.
- Need to declare an empty array to catch the results.
“Hey Jonathan, you don’t actually need to create a new empty array. Just modify the existing array!” I hear you argue.
Yep, you’re right! We don’t need to but you should. We could have done this.
var numbers = [1, 2, 3];
// We could do this, but try not to.
for( var i = 0; i < numbers.length; i++ ) {
numbers[i] = numbers[i] * 2;
}
In JavaScript applications, especially larger complex applications, we want to avoid mutating or changing data whenever possible. If we change the numbers in our numbers array, that would be changing or mutating data.
If we had been using the numbers array in other parts of our application we would start seeing weird bugs in those areas of the application since our numbers array which was [1,2,3] is now [2,4,6].
Anyway, I digress. Let’s see how the code would work with the map() helper.
var numbers = [1, 2, 3];
// New map helper.
var doubled = numbers.map(function(number) {
return number * 2;
});
console.log(doubled); // [2, 4, 6]
A bit more readable than the for loop, don’t you think?
Each number in the numbers array is being passed into the anonymous function and whatever this function returns, returns a new array. So with the map() helper we have two arrays. Our original array and we have a new array that was created from our map() helper.
The key point is that we’re not mutating the existing array. The map() helper is creating a brand new array.
It is worth noting that the return key used is necessary when using the map() helper. If you don’t return the result then it’s going to return null.
var doubled = numbers.map(function(number) {
number * 2;
});
console.log(doubled); // [null,null,null]
map() with Objects
Let’s try out a more complicated example with map(). This is something that you’ll see happen very frequently with JavaScript frameworks such as Angular and React.
You can make an array of cars and each car we’re going to say is going to be an object. The object is going to represent the different properties that a car might have.
var cars = [
{ model: 'Volkswagen', price: 'Cheap' },
{ model: 'Porsche', price: 'Expensive' }
];
One of the most common uses of map() that we’re going to be looking at is to collect properties off of an array of objects.
So let’s say that I want to iterate over my car’s array and I want to find the prices of each car in here.
I don’t really care about the model. I just want to kind of get a better idea of what are the different prices that I have for all the different objects in the array.
Let’s see how we might approach this by using the map() helper to make a new array called prices.
var cars = [
{ model: 'Volkswagen', price: 'Cheap' },
{ model: 'Porsche', price: 'Expensive' }
];
var prices = cars.map(function(car) {
return car.price;
});
console.log(prices); // [‘Cheap’, ‘Expensive’]
Now we have created a log of only the specific data we wanted, the prices of the cars.
This is what’s typically referred to as a pluck because we’re plucking a particular property off of each object in the array.
Continue Reading
-
CodePen Challenge: Bubbling
This month we explore concepts that help us get our animation juices flowing. We are also provided resources to help us learn GSAP or level up your GSAP skills.
-
CodePen Challenge: Handling User-Uploaded Images
In this week's Challenge, we start with a set of very different user-uploaded avatars and it's our job to do something with them to bring them together nicely.
-
JavaScript ES6: The Basics of Destructuring
Destructuring is one of the most common and important features of ES6. It allows us to extract data from arrays and objects, and assign that data into their own variables. Let's go over the basics!
-
CodePen Challenge: Gangnam Style
For this week's challenge, we make the shift from MTV to YouTube with the first video to get 1 billion views: "Gangnam Style" by Psy. Love it or hate it, if you were online in 2012 you definitely saw it. And you can't deny it's got some great colors!