React: Stateless Functional Components
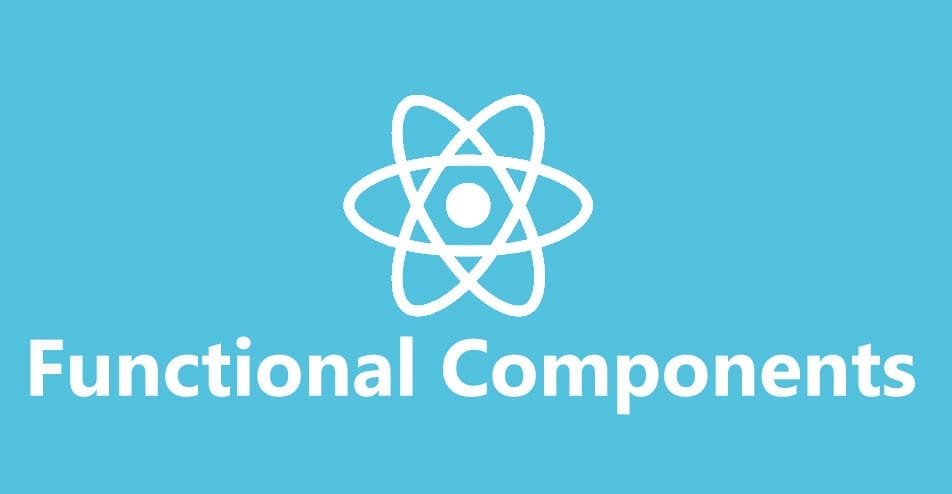
When creating React components, sometimes we find ourselves in a situation where we are creating large components just to output some HTML. This is usually overkill. Instead, we can use something called a Stateless Functional Component.
A Stateless Functional Component is a component that typically only contains a render method, which returns HTML, and prop types.
// Standard component
class Header extends React.Component {
render() {
return (
<header>
<h1 className="title">{this.props.title}</h1>
</header>
)
}
}
In the example above, we’re outputting a basic snippet of HTML with a single prop. There’s nothing wrong with writing it out that way but we end up writing a bunch of unnecessary code which can make it more difficult to read and bumps up our codes line count.
Follow the example above, first we’ll convert our component into ES5 and then refactor it to ES6.
// ES5
function Header(props) {
return (
<header>
<h1 className="title">{props.title}</h1>
</header>
)
}
An important change to not is on our prop. Since we are no longer using a Class to create the component, the keyword this is no longer used. Instead, we pass props into the function argument Header(props). Very handy for those who find the this keyword to be a bit confusing!
Great, we have our Stateless Functional Component working! There’s more we can do here to make it more readable and whittle down our code a bit.
First, we can turn our function into an arrow function.
// ES6 arrow function
const Header = (props) => {
return (
<header>
<h1 className="title">{props.title}</h1>
</header>
)
}
We can take this further with an implicit return by removing the need for the return keyword. This is possible since, by default, an arrow function will render a return on whatever appears within its parentheses.
// ES6 arrow function implicit return
const Header = (props) => (
<header>
<h1 className="title">{props.title}</h1>
</header>
);
We can take this EVEN FURTHER with Destructuring the props into their own variables. We can add curly braces in the function’s arguments with the name of our prop. Next, we can remove props. from where we print out the prop.
// ES6 Destructuring
const Header = ({ title }) => (
<header>
<h1 className="title">{title}</h1>
</header>
);
If you’re interested in some further reading about Destructuring in ES6, here’s an article I wrote on the basics.
Wrapping Things Up
Stateless Functional Components are a great way to improve our code readability, length, and performance if we know our component will only contain HTML and props.
A pitfall to watch out for would be that if you’re at the start of a project and you don’t know if that component will contain more than props and HTML then it’s best to use a regular component.
However, if you’re at the end of a project or you know for sure the component only needs HTML and props then Stateless Functional Component is the way to go!
Continue Reading
-
CodePen Challenge: Bubbling
This month we explore concepts that help us get our animation juices flowing. We are also provided resources to help us learn GSAP or level up your GSAP skills.
-
JavaScript ES6: The forEach() Helper
forEach() is an ES6 helper that is used to call a function once on each item in an array and is arguably the most useful ES6 helper. Let's dig into how it works.
-
Next-gen Images: Page Speed’s New Best Friend
Converting your images to a next-gen image format, like WebP, is one of the best ways to improve the user’s experience and page speed on your website.
-
Understanding SSL Certificates
SSL certificates are not optional any more, they are critical. Here we help you get a better understanding of what exactly they provide.