JavaScript ES6: The forEach() Helper
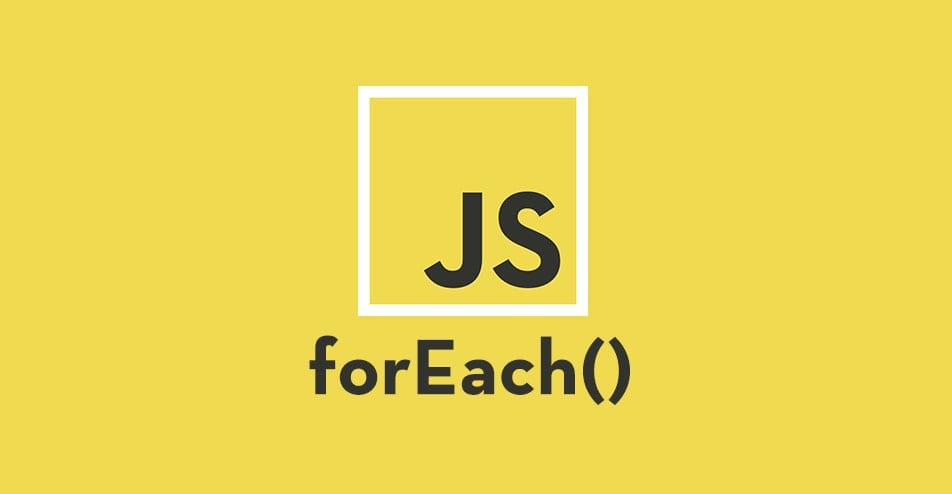
forEach() is an ES6 helper that is used to call a function once on each item in an array. It’s iteration over an array or list.
In ES5, if we wanted to iterate over an array, we would make use of a for loop.
var colors = [ 'red', 'blue', 'green' ];
for( var i = 0; i < colors.length; i++) {
console.log(colors[i]);
}
The ES5 for loop works fine. It will go through each item in an array and console log it but if an error occurs it can be challenging to see what’s going wrong, especially if there is lots of logic to follow.
ES6 introduces the forEach() helper which can replace the for loop. But why bother doing it a different way? Well, I find the for loop has some disadvantages:
- Lots of different elements of code.
- Prone to typos.
- Semicolons are between each element which is uncommon in JavaScript syntax.
- The more logic in a single line of code the more difficult it is for future developers to understand what’s going on.
Now let’s see how the ES6 forEach() helper improves on the for loop.
var colors = [ 'red', 'blue', 'green' ];
colors.forEach(function(color) {
console.log(color);
});
Ah, much cleaner! The forEach() helper improves on the for loop in a few ways:
- Uses less code.
- Less logic to get wrong.
- Easier to read.
- Removes the need of an iterator variable.
How forEach() Works
forEach() is an array helper method. When we call it we pass in an anonymous function which is the internal argument to the forEach() call.
The function gets called one time for each item in the array and whatever is in the function, happens.
// 1. Create an array of numbers.
var numbers = [1, 2, 3, 4, 5];
// 2. Create a variable to hold the sum.
var sum = 0;
// 3. Loop over the array, incrementing the sum variable.
numbers.forEach(function(number) {
sum += number;
});
// 4. print the sum variable.
console.log(sum);
Common convention is that in your array, you call it as a plural. So if we have an array containing numbers, we call the array “numbers”.
In your iterator function, when we receive an individual element in that array then we’ll use the singular. In our “numbers” array we’ll use “number” in the iterator function.
The function in the forEach helper doesn’t have to be anonymous. We can declare it separately and then pass it into forEach.
// 1. Create an array of numbers.
var numbers = [1, 2, 3, 4, 5];
// 2. Create a variable to hold the sum.
var sum = 0;
// 3. Create our adder function
function adder(number) {
sum += number;
}
// 4. Loop over the array, incrementing the sum variable except this time a function gets referenced in the forEach
numbers.forEach(adder);
// 5. print the sum variable.
console.log(sum);
NOTE: When adding a function into the iterator we don’t use parentheses.
Conclusion
The ES6 forEach() is arguably the most useful ES6 helper. A lot of the other ES6 helpers could be reimplemented using forEach().
Whenever you want to call a function multiple times passing in a different argument each time, for each item in an array or list, we use the forEach() helper.
Continue Reading
-
ACF Relationship Field Prev/Next Buttons
Looking to create custom previous and next buttons from ACF's Relationship field with the full code snippet provided? Then you've come to the right place!
-
Using robots.txt to prevent staging sites from indexing
Going over the basics of the robots.txt and the solution to prevent your staging site from being indexed.
-
Web Accessibility & Why its Crucial in 2021
Web accessibility is all about inclusivity. It’s the idea that everyone, regardless of limitations, should have the same opportunity to view content on the web like everyone else. These limitations include visual, auditory or physical disabilities. Making your website accessible will become ever more crucial in 2021.
-
CodePen Challenge: Handling User-Uploaded Images
In this week's Challenge, we start with a set of very different user-uploaded avatars and it's our job to do something with them to bring them together nicely.